- EP 3 - [C++] Polymorphism, Function overriding and Virtual function.
🚥 polymorphism is the occurrence of two or more different morphs or forms.🚥
In this episode, I am talking about Polymorphism and more specifically Runtime polymorphism. This episode is arranged in such a way that it will help you to understand the concept clearly. There are a few common interview questions and I am explaining the answer in the best possible way.
You can read the transcript below.
This is very important and frequently asked interview questions.
Don't forget to subscribe to the podcast to get a notification.
You can listen on
➡️ Amazon Music (https://music.amazon.com/podcasts/c5ed5ab6-e4e8-4932-a397-b687c85465da/PROGRAMMING-GYAN)
➡️ Spotify (Programming Gyan)
➡️ Apple Podcast (https://podcasts.apple.com/us/podcast/programming-gyan/id1568920481)
➡️ Stitcher (https://www.stitcher.com/podcast/programminggyan)
➡️ Google Podcast (https://podcasts.google.com/feed/aHR0cHM6Ly9mZWVkcy5yZWRjaXJjbGUuY29tL2JiOWQxMjEzLTFjODctNGRmZS1hNjZhLTIyM2MxNTc5NTE2OQ)
You have lots of options. So there is no reason not to listen.
You can follow #programminggyan
LinkedIn: ProgrammingGyan (https://www.linkedin.com/company/programminggyan)
Twitter: @GyanProgramming (https://twitter.com/GyanProgramming)
Instagram: @programming_gyan (https://www.instagram.com/programming_gyan)
Facebook: @ProgrammingGyan (https://www.facebook.com/ProgrammingGyan)
To know more about me and to read blogs visit www.jhadheeraj.com
Follow me at
#LinkedIn: @jhadheeraj (https://www.linkedin.com/in/jhadheeraj)
#Twitter: @dheerajjha_03 (https://twitter.com/dheerajjha_03)
#Instagram: @jha.dheeraj03 (https://www.instagram.com/jha.dheeraj03/
-------------------------------------------------------------------------------------------
Transcript:
Welcome to Programming Gyan [C++]- Tips and Tricks with Dheeraj Jha
EP 3 - Polymorphism, Function overriding, and Virtual function.
C++ is an object-oriented programming language. It supports all the OOP concepts. Today I am going to talk about one of the OOP concepts. Polymorphism.
In biology, polymorphism is the occurrence of two or more different morphs or forms. It is the same in C++.
Polymorphism in programming means the same name with a different implementation. This allows us to do an operation in many ways based on the input.
In C++, you have two types of polymorphism: Overloading and Overriding.
In this episode, I will talk about Overriding. And will talk about Overloading in another episode. It is important to know the use case where you can use the tool. The problem why we need a tool. I will talk in a format so that you will get to know all the things in sequence. This episode will also help you to prepare for common interview questions.
- What is function overriding?
Inheritance allows us to create derived classes from the base class and inherit features of the base class. When we create a derived class object and call a function defined in the base class, the base class function gets called. If we redefine this function of the base class in the derived class, we can call the derived class function using the derived class object.
So what actually happened here is called Function Overriding.
Code ref: https://godbolt.org/z/f4Wfbqcxn
Remember here, function overriding is only possible when there is inheritance.
- Why we need a virtual function?
- In what scenario we use the virtual function?
Now when we are talking about function overriding, it is important to talk about Virtual functions. So before we go deep into internals, let's understand why we need this? In which scenarios we can use this feature of C++?
Consider you are developing an automotive application which deals with different types of vehicles. So you created a base vehicle class and derived different derived classes like a car, bike, etc. For simplicity, I will use the car and bike-derived classes.
All the vehicles have some common high-level features like start, stop, accelerate, etc. So you added these functions in the base class and overridden them in derived classes. And you started using your derived classes with their own implementation. Everything is going well so far.
You were enjoying your code and someday, you got a new requirement that the user will decide which vehicle he is using and your application should work as per the user's vehicle.
Oh…
Now you don’t know which vehicle the user owns when you are writing your code. The type of vehicle will be decided at runtime when a user is using the application.
To solve this you can check the type of vehicle user is providing at run time and call the appropriate function for the vehicle type. But this is not a good solution as your application may support more types of vehicles in the future.
Here comes the virtual function for rescue.
Code to refer: https://godbolt.org/z/ebc1Pn5eP
- How virtual function works?
In the base class, you write a Virtual keyword before the function declaration to create a virtual function. When you create a virtual function in your class. There are a few things compiler does for you.
- The compiler will add VTable for the class which has a virtual function.
- Vtable is used by the compiler to keep track of virtual functions associated with the class.
- There is only one instance of Vtable for each class and all the instances or objects of the class will point to this vtable.
- Your C++ compiler adds function pointers in Vtable which will point to the virtual function which needs to be called. In short, Vtable stores function pointers of virtual functions.
- When you instantiate an object of this class, the compiler will also add a pointer to the object. This pointer is usually referred to as vptr. When you instantiate an object, in the constructor this vptr pointer is initialized and it points to Vtable. All these things happen implicitly for you. So you need not write any extra code for this.
- Remember a point here, if your class has a pure virtual function, there will be no entry in Vtable for pure virtual function as the pure virtual function doesn't have a definition. Although you can provide a definition of pure virtual function which I will discuss in a future episode.
Before I move ahead, I want to mention here that the C++ standards do not mandate exactly how dynamic dispatch must be implemented. Compilers generally use minor variations on the same basic model.
Now when you derive a class from the base class with virtual function,
a new Vtable is created for the derived class
- It maintains the offsets of base class vtable
- If you have any overridden virtual function in the derived class, the entry in vtable will be replaced by a derived class function definition.
- If any base class virtual function is not overridden, its entry will be preserved.
I hope you are clear now what happens when you create a virtual function in a class.
Now let's consider the problem scenario I explained before.
You will create a base vehicle class pointer that can point to any of the derived class objects.
Vehicle *vehicleptr = nullptr;
Now you can pass any object of the derived class and assign it to this vehicle pointer.
You can now call vehicleptr->start(), vehicleptr->accelerate(), vehicleptr->stop().
When you call any virtual function using a base class pointer, it checks in the vtable of the object, vehicleptr is pointing to. Calls respective function definition from the vtable and execute.
I hope you can visualize here that now you don't have to worry about the user input. Whatever vehicle type object, the user is passing, your code will work as per that.
I will add all the reference code links in the description, do check out those.
There are few interview questions on this topic which I will discuss in a future episode.
I hope, you got something new from this episode. Subscribe to this podcast and follow me on LinkedIn, Twitter, and Instagram. I will add links in the description. To know more about me you can visit www.jhadheeraj.com
I will meet you in the next episode with some other interesting tips and tricks.
Thank you for listening ProgrammingGyan [C++] -Tips and Tricks with Dheeraj Jha.
S1E3 - 8m - Jun 21, 2021 - Ep 2 - [C++] What happens when you write an Inline function?
Inline is a request to the compiler, not a command
Inline function is an important concept in C++ and it is a widely asked interview question also.
In this episode, Dheeraj is talking about the Inline function. He talks about how to create an Inline function. What the compiler does when you write an inline function?
There is a bonus tip in the episode. So keep listening.
LinkedIn: https://www.linkedin.com/in/jhadheeraj
Twitter: https://twitter.com/dheerajjha_03
Instagram: https://www.instagram.com/jha.dheeraj03
-------------------------------------------------------------------------------------------
Transcript:
Welcome to Programming Gyan - Tips and Tricks with Dheeraj Jha
Episode2 - [C++] What happens when you write an Inline function?
In episode one when I was discussing the macro functions or you can say function-like macros, I suggested you use inline functions instead. I also gave you some brief about the inline function.
I thought it’s a good topic to talk about in 2nd episode. In this episode, I will give you some more details of inline functions. When you should use it and when not. I will also tell you how the compiler handles inline functions.
How to write an inline function?
So before we go into details, let's see what are the ways to define inline function.
when you define a non-class member inline function,
- The Declaration of an inline function is like a normal function.
- you prepend the function’s definition with the keyword inline, and you put the definition into a header file.
You can also define a class member inline function. Steps are same
- The declaration is the same as other member functions.
- You will have to add an inline keyword before the definition of your inline function.
- And define your function outside the class body but in the header file.
There is another way to create a class member inline function.
- You define your function within your class definition where you are declaring your function.
- No need to use the Inline prefix.
I will ask you a question here.
Do you have any idea Why you should define your inline function in the header file?
It's fine if you don't know the answer and I will answer this question in the end.
But what actually happens when you define an Inline function?
When you define a function, it gets stored in the code segment of your application.
Now when you call this function in your program, your CPU loads this function executes and returns to the place from where this function gets called, to the caller.
When you define a function as inline, the compiler adds the function definition where the function is getting called. By doing this you save the cost of a function call.
In real life, there is no free lunch. Inline functions are also not exceptions. Inline functions save the function call costs by replacing the function call with the function body. I hope you must have observed here that it will increase the object code size.
And if in excitement you write lots of inline functions, it's not good for the machines which run on low or limited memory or many embedded systems.
Your compiler does all this for you. But remember this, Inline is a request to the compiler, not a command. Adding the Inline keyword doesn't guarantee this optimization.
The compiler can ignore the request for inline. The compiler may not perform inline in such circumstances like:
- If a function contains a loop, switch, or goto statement or it contains static variables.
- The compiler ignores recursive functions for this optimization.
- Virtual functions defy inlining. This should not surprise as virtual means "wait till runtime" and inline is "before execution".
Remember sometimes compiler generates a function body for a function that is a perfect candidate for inlining. Now the question is why? I can't give you all the answers here but will give you some idea.
Consider you have a function pointer that is pointing to your function, which is a good candidate for inlining. And also you added the Inline keyword. Now because your function is inline(assume compiler did required optimizations), it will not get memory in the code segment. Now think about what will happen when you call your function using a function pointer.
Intelligently compiler refuses your request in such cases and saves you.
I hope till now you are with me and understood how Inline works.
Now the question comes to do Inline functions improve performance?
There is no straight yes or no. You will have to play with it to see what suits you best in your case.
Do not stick to any answer like "Never use" or "Always use Inline function" or someone says "Use inline functions if it is less than N number of lines." You can use these as guidelines but don't rely on them.
Now coming back to the question, I asked you Do you have an idea Why you should define your inline function in the header file?
Inline functions should be in header files because most build environments do inlining during compilation. To replace the function call with the function body, the compiler needs to know how the function looks like.
If you put the inline function’s definition into a .cpp file and you call it from some other .cpp file, you’ll get an “unresolved external” error from the linker. You can still provide the definition in the .cpp file till you use that function in the same file.
I hope, you got something new from this episode. Subscribe to this podcast and follow me on LinkedIn, Twitter, and Instagram. I will add links in the description. To know more about me you can visit www.jhadheeraj.com
I will meet you in the next episode with some other interesting tips and tricks.
Thank you for listening ProgrammingGyan-Tips and Tricks with Dheeraj Jha.
S1E2 - 6m - Jun 6, 2021 - Ep 1 - [C++] Prefer const and inline to #define
Prefer the compiler to the preprocessor.
In this episode, Dheeraj is talking about the drawbacks of using #define and what are the better alternate in different cases
Listen to more episode at www.jhadheeraj.com/podcast
To know more about me and to read blogs visit www.jhadheeraj.com
Follow me at
LinkedIn: https://www.linkedin.com/in/jhadheeraj/
Twitter: @dheerajjha_03
Instagram: jha.dheeraj03
Ref:
Effective C++ by Scott Meyers
S1E1 - 5m - May 26, 2021 - Intro to ProgrammingGyan
ProgrammingGyan is mainly for people interested in programming and software development.
Here you will learn lots of tips and tricks in programming, best practices and lot more.
1m - May 21, 2021
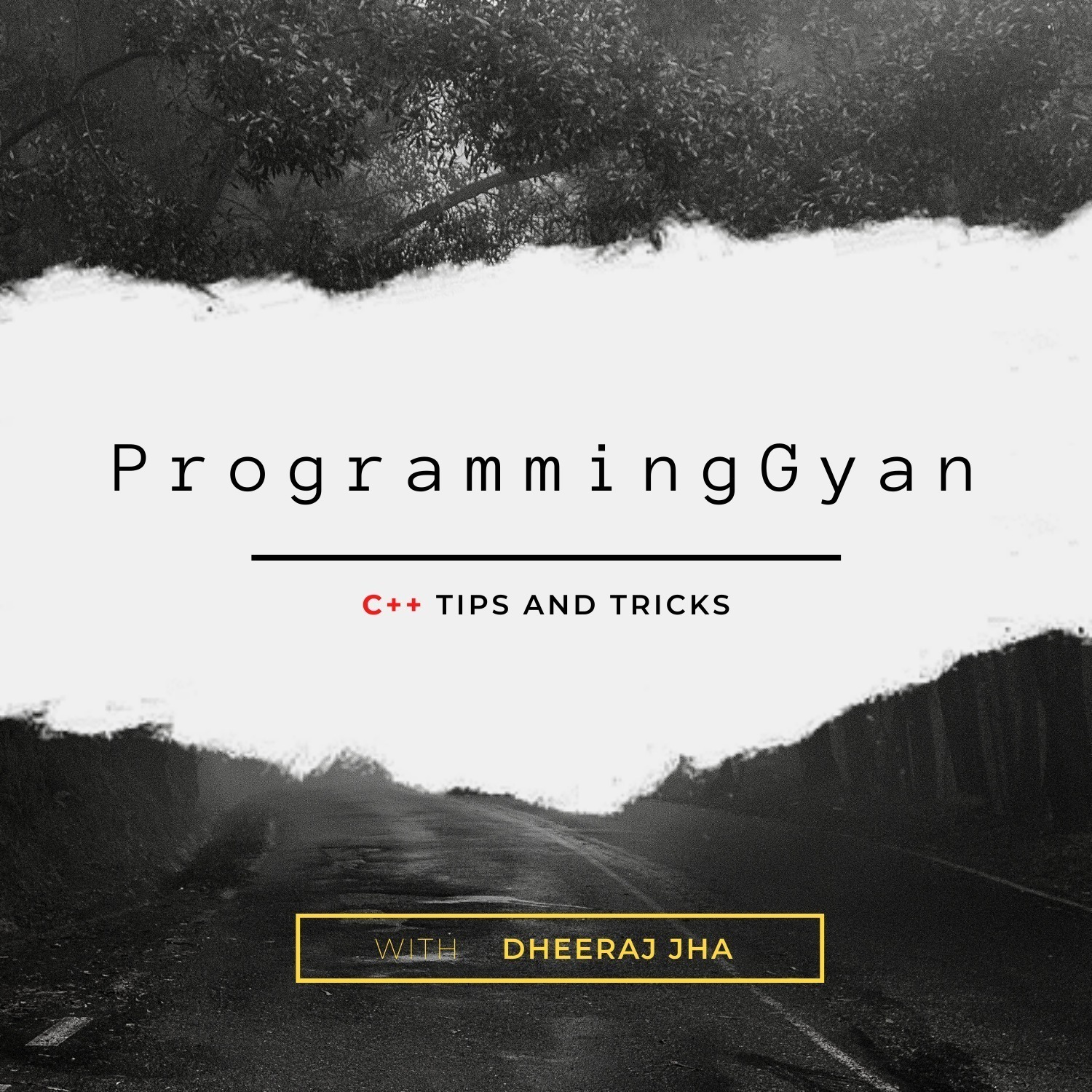